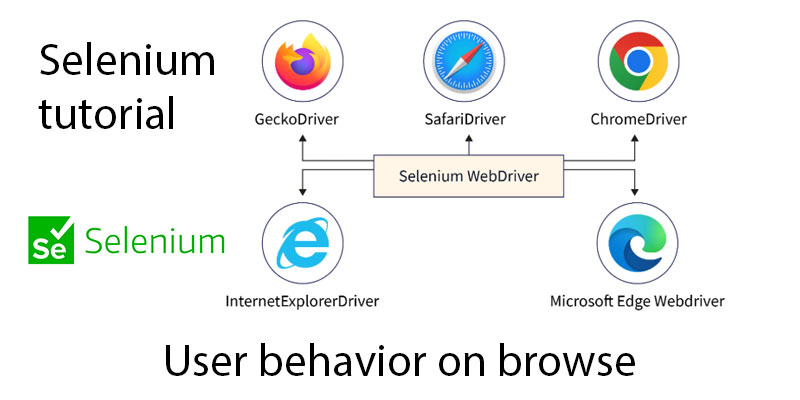
Selenium provides a way to record and playback user interactions on web browsers, allowing developers to test the
functionality of their applications. Here’s an overview of user behavior on browser in Selenium:
Recording User Interactions
When you use Selenium to interact with a web application, it records the following user behaviors:
- Mouse Events: Clicks, double clicks, right-clicks, drag-and-drop, and mouse movements.
- Keyboard Input: Typing characters, sending keyboard events (e.g., enter, tab).
- Navigation: Page navigation (e.g., clicking links, clicking buttons), back/forward browsing.
- Form Interactions: Filling out forms, submitting forms, selecting options from dropdowns.
Playback of Recorded User Behaviors
When you playback the recorded user interactions using Selenium, it simulates these behaviors on the target web
application. This allows developers to test their applications under different scenarios and identify potential
issues.
Example Use Cases:
- Automated Testing: Record user interactions for a web application and playback them using Selenium to
ensure its functionality. - Browser Scripting: Record and playback user interactions for a browser automation project, such as a script
that simulates user behavior on a website. - Web Scraping: Record user interactions while extracting data from a web page, allowing you to test the
stability of your scraper.
Some examples of common actions
Open Browser
from selenium import webdriver
driver = webdriver.Chrome() # Initialize Chrome browser
driver.get("http://example.com") # Open a website
Close Browser
driver.quit() # Close the entire browser
Maximize Window
driver.maximize_window() # Maximize the window
Navigate to URL:
driver.get("http://example.com") # Navigate to a different URL
Refresh Page
driver.refresh() # Refresh the current page
Back and Forward
driver.back() # Go back to the previous page
driver.forward() # Go forward to the next page
Get Title
- To retrieve the title of the current page, you can use the
title
property or a method provided by Selenium’s WebDriver.
# Assuming 'driver' is your WebDriver instance
title = driver.title # Get the title of the current page
print(title) # Print the title to the console
Get Source Code
- To retrieve the source code (HTML content) of the current page, you can use the
page_source
property provided by Selenium’s WebDriver.
# Assuming 'driver' is your WebDriver instance
source_code = driver.page_source # Get the source code of the current page
print(source_code) # Print the source code to the console
Handling Alerts (also known as pop-ups)
- When an alert appears in the browser, you can handle it using Selenium’s built-in methods for accepting or dismissing it.
from selenium import webdriver
from selenium.webdriver.common.alert import Alert
driver = webdriver.Chrome()
driver.get("http://example.com")
# Switch to the alert and accept it
alert = Alert(driver)
alert.accept() # Accepts the alert
# or dismiss it
alert.dismiss() # Dismisses the alert
Handling Windows/Tabs
- You can switch between windows or tabs using WebDriver commands. Each window or tab is represented by a unique handle, which you can manage through Selenium’s methods.
driver = webdriver.Chrome()
driver.get("http://example.com")
# Open a new tab (or window) and navigate to another URL
driver.execute_script("window.open('http://another-example.com', '_blank');")
# Switch to the new tab/window by its index (0 is the original tab)
driver.switch_to.window(driver.window_handles[1])
Handling Frames
- To interact with elements inside frames or iframes, you need to switch the context to the frame first.
# Switch to an iframe by its name or ID
driver.switch_to.frame("iframe-name") # Replace 'iframe-name' with the actual name or ID of the iframe
# To switch back to the main content after interacting with the iframe, use:
driver.switch_to.default_content()
Note
- args0 = argument 1 (tham số ở vị trí đầu tiên)
- Các hàm bắt đầu bằng get thì thường sẽ trả về kiểu dữ liệu
Best Practices:
- Use Explicit Waiting: Use explicit waiting mechanisms (e.g., WebDriverWait) to wait for specific elements
or conditions to be present before proceeding with playback. - Avoid Over-Loading the Browser: Avoid over-loading the browser with too many interactions in a short
period, as this can slow down your test or cause issues. - Use Proper Data Handling: Handle user input data correctly and securely, using methods like parameterized
testing to avoid hardcoding sensitive information.
By recording and playing back user interactions on browsers using Selenium, you can create robust automated tests
that simulate real-world scenarios and help ensure the stability of your web applications.